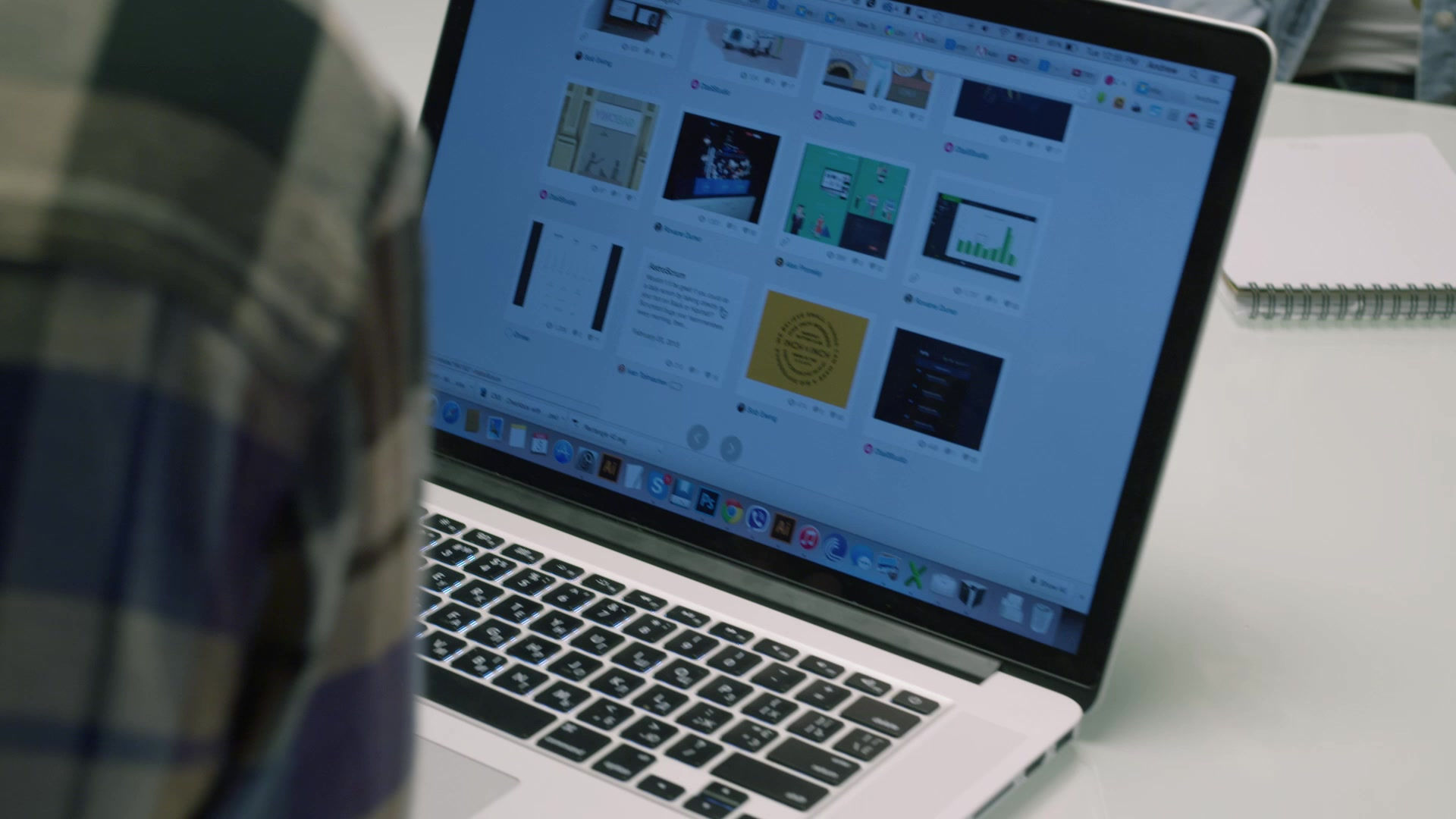
Write a program (in the language of your choice) to implement a directory of a set of
student records by using open addressing. Your program should take its input from a text file
“namal.txt”, which is stored in the same folder as that of your .java file.
Open the following file for complete problem statement and its solution.
some problems solved in java
Insertion in arrays!
1. Write a function for the insertion at first index of an Array.
2. Write a function for the insertion at last index of an Array.
3. Write a function for the insertion at desired index of an Array.
Solution is attached below.
Queue And DeQue by arrays and linkedlist!
1. implement DeQue by arrays!
2. implement QDeQue by linkedlist!
3. implement Queue by arrays!
4. implement Queue by linkedlist!
5. implement stack by arrays!
6. implement stack by linkedlist!
Solution is attached below.
Find the desired number in arrays
-
Let S be an unsorted array of n integers. Give an algorithm that finds the pair
x, y ∈ S that maximizes |x − y |. -
Let S be a sorted array of n integers. Give an algorithm that finds the pair
x, y ∈ S that maximizes |x − y |. -
Let S be an unsorted array of n integers. Give an algorithm that finds the pair
x, y ∈ S that minimizes |x − y |, for x = y.
Solution is attached below.
Mod of set of n numbers
-
The mode of a set of numbers is the number that occurs most frequently in the set. The set {4, 6, 2, 4, 3, 1} has a mode of 4. Give an efficient and correct algorithm
to compute the mode of a set of n numbers. You have to take numbers as input.
Solution is attached below.
Remove duplicate elements
-
Write a Java program to remove the duplicate elements of a given array and return the new length of the array. Sample array: [20, 20, 30, 40, 50, 50, 50]
After removing the duplicate elements the program should return 4 as the new length of the array.
Solution is attached below.
Print all leaders
-
Write a Java program to print all the LEADERS in the array[An element is leader if it is greater than all the elements to its right side.]
Solution is attached below.
Sum with recursive function
-
Write a recursive function that computes the sum of all numbers from 1 to n, where n is given as parameter
Solution is attached below.
Recursive function examples
-
Write a recursive function that finds and returns the minimum element in an array, where the array and its size are given as parameters.
-
Write a recursive function that computes and returns the sum of all elements in an array, where the array and its size are given as parameters.
Solution is attached below.
Transfer stack function
-
Write a function transferStack (Stack one, Stack two): It transfers all elements in the first stack into the second stack, keeping the same order. So what was initially on top of one ends up on top of two.
Solution is attached below.
Binary search tree
-
Write a Java program to create a Binary Search Tree. This Binary Search Tree is to store the integer values. Your program should display a menu of choices to operate the Binary Search Tree data structure. See the sample menu below:
-
1.Add a node 2.Delete a node 3.Search a value
2, Print all elements of binary tree using following tree traversal techniques discussed in class.
-
Post-order Traversal
-
In-order Traversal
-
Pre-order Traversal